Sorting challenge
Posted on 25/10/07 by Felix Geisendörfer
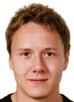
Quick challenge,
lets say you have an array like this:
array('Product' => array('ordering' => 5))
, array('Product' => array('ordering' => 3))
, array('Product' => array('ordering' => 9))
, array('Product' => array('ordering' => 1))
);
and you want to iterate through your products by Product.ordering ASC. Whats the fastest way to do this? I just came up with this nifty little attempt, but I'd love to see some other solutions. (Rules: Don't use anything eval()'ish, but anything you find in Cake 1.2 can be used).
ksort($order);
while ($product = $products[array_shift($order)]) {
debug($product);
}
-- Felix Geisendörfer aka the_undefined
You can skip to the end and add a comment.
jickles: Hm, but that wouldn't work if there are user keys like Product.id in the array? I mean is there a way to specify what key you want to sort by using array_multisort?
@Felix:
I think array_multisort could be used like this:
$products = array(
array('Product' => array('ordering' => 5, 'id' => 2))
, array('Product' => array('ordering' => 9, 'id' => 9))
, array('Product' => array('ordering' => 1, 'id' => 11))
, array('Product' => array('ordering' => 5, 'id' => 3))
);
foreach ($products as $key => $val ) {
$ordering[$key] = $val['Product']['ordering'];
$id[$key] = $val['Product']['id'];
}
array_multisort($ordering, SORT_ASC, $id, SORT_ASC, $products);
print_r($products);
Also you can put type of sort, because some time you have to sort strings, another time you have to sort numbers. so the example above could be modified slightly and let's imagine that we have some name istead of ordering:
Example
$products = array(
array(’Product’ => array(’name’ => 'Matias', ‘id’ => 2))
, array(’Product’ => array(’name’ => 'Felix', ‘id’ => 9))
, array(’Product’ => array(’name’ => 'Jackles', ‘id’ => 11))
, array(’Product’ => array(’name’ => 'Nik', ‘id’ => 3))
);
array_multisort($name, SORT_ASC, SORT_STRING, $id, SORT_ASC, SORT_NUMERIC, $products);
print_r($products);
I can say that I've did a class in the past which sorts the multiple arrays for Html table presentation element and so far it still works as expected without any problem. ;)
$b) ? -1 : 1;
}
usort($products, 'sortProducts');
print_r($products);
?>
Wow, my previous post got munged. I'll try without the PHP tags.
function sortProducts($a, $b) {
$a = $a['Product']['ordering'];
$b = $b['Product']['ordering'];
if ($a == $b) return 0;
return ($a
Felix Geisendorfer's Blog: Sorting Challenge...
...
Oh my .. I have no idea how to post code here, maybe convert it to HTML? ..
Well, my solution is here: http://davidp.dk/sorting.php
I would also do it the "usort" way.
[...] Felix Geisendorfer has a quick little sorting example posted today showing on way to sort a multi-dimensional array. Quick challenge, lets say you have an array and you want to iterate through your products by [the key of each subarray in $products] Product.ordering ASC. Whats the fastest way to do this? [...]
@everybody: You can paste code here: http://bin.cakephp.org/add and then post the link.
Thanks for the solutions provided so far, I'm wondering how my Set::extract / flip / sort mashup would do performance wise. The uSort solution might be the fastest.
[...] Felix Geisendorfer’s Blog: Sorting Challenge Posted in October 27th, 2007 by admin in News, PHP News Felix Geisendorfer has a quick little sorting example posted today showing on way to sort a multi-dimensional array. Quick challenge, lets say you have an array and you want to iterate through your products by [the key of each subarray in $products] Product.ordering ASC. Whats the fastest way to do this? [...]
This post is too old. We do not allow comments here anymore in order to fight spam. If you have real feedback or questions for the post, please contact us.
How about this:
array_multisort($products, SORT_ASC);
foreach($products as $product)
debug($product);
I think it works.
jickles